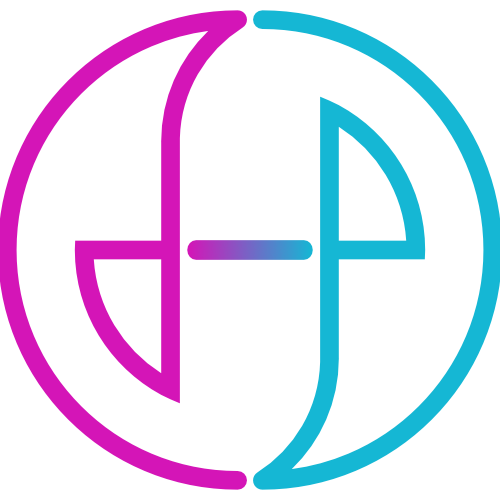

Sharing States Between Machines in xState react
State management is a crucial aspect of modern web development, and xstate is a powerful library that can help you manage the state of your React applications. One of the key features of xstate is the ability to share state between different state machines, which can help you create more complex and dynamic applications.
In this blog post, we will explore how to share state between state machines in xstate-react. We will start by creating two state machines, Machine A and Machine B, and then we will show how to use the xstate.assign
method to share state between these machines.
First, let's create Machine A:
import { Machine } from 'xstate';
const machineA = Machine({
id: 'machineA',
initial: 'idle',
states: {
idle: {
on: {
START: 'running',
},
},
running: {
on: {
STOP: 'idle',
},
},
},
});
Machine A has two states, idle
and running
, and two events, START
and STOP
. When the START
event is sent, Machine A will transition from the idle
state to the running
state. Similarly, when the STOP
event is sent, Machine A will transition from the running
state to the idle
state.
Next, let's create Machine B:
const machineB = Machine({
id: 'machineB',
initial: 'waiting',
states: {
waiting: {
on: {
START: {
target: 'running',
actions: assign({
machineA: (context, event) => machineA.transition(event.type),
}),
},
},
},
running: {
on: {
STOP: {
target: 'waiting',
actions: assign({
machineA: (context, event) => machineA.transition(event.type),
}),
},
},
},
},
});
Like Machine A, Machine B also has two states, waiting
and running
, and two events, START
and STOP
. However, in this machine, when the START
event is sent, it will transition to the running
state and update the state of Machine A to running
. Similarly, when the STOP
event is sent to machineB
, it will transition to the waiting
state and update the state of Machine A to idle
.
This is done by using the xstate.assign
method inside the on
property of a state. The assign
method allows you to update the state of one machine based on the state of another machine. In the above example, we are using the machineA.transition(event.type)
function to update the state of Machine A based on the event that is sent to Machine B.
Finally, you can use xstate.useMachine
hook to connect machineA and machineB to react component.
function MyComponent() {
const [stateA, sendA] = useMachine(machineA);
const [stateB, sendB] = useMachine(machineB);
return (
<div>
<div>
<button onClick={() => sendA("START")}>Start A</button>
<button onClick={() => sendA("STOP")}>Stop A</button>
<button onClick={() => sendB("START")}>Start B</button>
<button onClick={() => sendB("STOP")}>Stop B</button>
</div>
</div>
);
}
In this way, the state of Machine A and Machine B is always in sync, and you can use the state of one machine to control the behavior of the other machine.
In conclusion, xstate provides a powerful and flexible way to share state between state machines in your React applications. By using the xstate.assign
method, you can easily update the state of one machine based on the state of another machine, allowing you to create more complex and dynamic applications.
In summary, xstate is a powerful library for managing state in React applications. The xstate.assign
method allows you to share state between different state machines by updating the state of one machine based on the state of another machine. This allows you to create more complex and dynamic applications by using the state of one machine to control the behavior of the other machine. To share state between state machines in xstate-react, you need to first create two state machines, Machine A and Machine B, then use the xstate.assign
method inside the on
property of a state in Machine B to update the state of Machine A based on the event that is sent to Machine B, and finally use the xstate.useMachine
hook to connect Machine A and Machine B to react component.
Discussion (1)
Helpful?
2
Jelly Fish
2023-02-02
Great article
1